Local Broadcast, less overhead and secure in Android
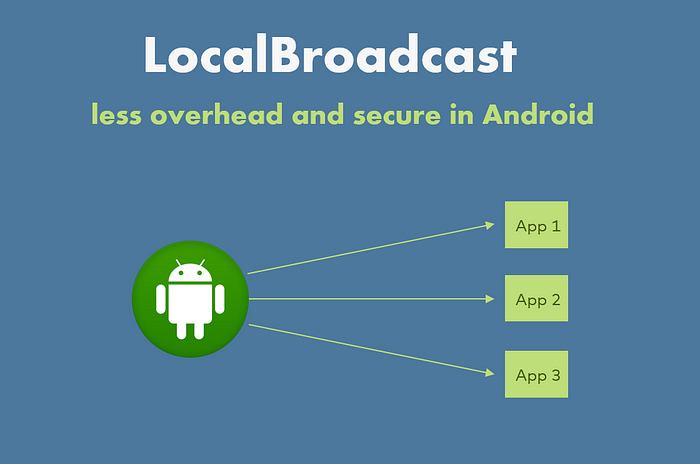
Broadcast receiver is an Android component which allows you to send or receive Android system or application events. All the registered application are notified by the Android runtime once event happens.
It works similar to the publish-subscribe design pattern and used for asynchronous inter-process communication.
For example, applications can register for various system events like boot complete or battery low, and Android system sends broadcast when specific event occur. Any application can also create its own custom broadcasts.
Basics of Broadcast
Let’s discuss some basic concepts of broadcast receiver.
Register Broadcast
There are two ways to register broadcast receiver-
Manifest-declared (Statically) : By this receiver can be registered via the AndroidManifest.xml file.
Context-registered (Dynamically) : By this register a receiver dynamically via the Context.registerReceiver() method.
Receive Broadcasts
To be able to receive a broadcast, application have to extends the BroadcastReceiver abstract class and override its onReceive() method.
If the event for which the broadcast receiver has registered happens, the onReceive() method of the receiver is called by the Android system.
Problem with global broadcast
It is good practice to use broadcast receivers when you want to send or receive data between different applications. But if the communication is limited to your application then it is not good to use the global broadcast.
In this case Android provides local broadcasts with the LocalBroadcastManager class. Using global broadcast, any other application can also send and receives broadcast messages to and from our application. This can be a serious security thread for our application. Also global broadcast is sent system-wide, so it is not performance efficient.
What is LocalBroadcastManager?
For obvious reasons, global broadcasts must never contain sensitive information. You can, however, broadcast such information locally using the LocalBroadcastManager class, which is a part of the Android Support Library.
The LocalBroadcastManager is much more efficient as it doesn’t need inter-process communication.
Below are some of its benefits:
- Broadcast data won’t leave your app, so don’t need to worry about leaking private data.
- It is not possible for other applications to send these broadcasts to your app, so you don’t need to worry about having security holes they can exploit.
- It is more efficient than sending a global broadcast through the system.
- No overhead of system-wide broadcast.
Implementation
There is no additional support library dependency required in the latest version of Android Studio. However, if you want to implement local broadcasts in an old project, following dependency needs to be add in the app module’s build.gradle file:
compile ‘com.android.support:support-v4:23.4.0’
Create a new instance of the LocalBroadcastManager
LocalBroadcastManager localBroadcastManager = LocalBroadcastManager.getInstance(context);
You can now send local broadcasts using the sendBroadcast() method like
// Create intent with actionIntent localIntent = new Intent(“CUSTOM_ACTION”);// Send local broadcastlocalBroadcastManager.sendBroadcast(localIntent);
Now create a broadcast receiver that can respond to the local-broadcast action:
private BroadcastReceiver listener = new BroadcastReceiver() {@Override public void onReceive( Context context, Intent intent ) { String data = intent.getStringExtra(“DATA”); Log.d( “Received data : “, data); }};
Dynamically registered receivers must be unregistered when they are no longer necessary like:
localBroadcastManager.unregisterReceiver(myBroadcastReceiver);
You can find the reference code to implement Local Broadcast receiver from GitHub. In the sample code I have created an IntentService, which broadcasts current date and it is received by an Activity of the same application.
How to secure broadcasts
Restrict your app to receive broadcast
- Specify a permission parameter when registering a broadcast receiver then only broadcasters who have requested the permission can send an Intent to the receiver.
For example, receiving app has a declared SEND_SMS permission in the receiver as shown below:
<receiver android:name=”.MyBroadcastReceiver” android:permission=”android.permission.SEND_SMS”> <intent-filter> <action android:name=”android.intent.action.AIRPLANE_MODE”/> </intent-filter></receiver>
- Set the android:exported attribute to “false” in the manifest. This restrict to receive broadcasts from sources outside of the app.
- Limit yourself to only local broadcasts with LocalBroadcastManager.
Control receiver of your broadcast
- You can specify a permission when sending a broadcast then only receivers who have requested that permission can receive the broadcast. For example, the following code sends a broadcast:
sendBroadcast(new Intent(“com.example.NOTIFY”), Manifest.permission.SEND_SMS);
- In Android 4.0 and higher, you can specify a package with setPackage(String) when sending a broadcast. The system restricts the broadcast to the set of apps that match the package.
- Send local broadcasts with LocalBroadcastManager.
Conclusion
Hope you understand about the global and local broadcasts and their security consideration. To improve the performance of system Android O has changed the registration of broadcast receiver. From Android O you cannot register implicit broadcasts in your application manifest (few exceptions is there) but application can continue to register for explicit broadcasts in their manifests or at run-time. Read more on broadcast receiver at Android official document.
Thanks for reading. To help others please click ❤ to recommend this article if you found it helpful. Stay tuned for upcoming articles. For any quires or suggestions, feel free to hit me on Twitter Google+ LinkedIn
Check out my blogger page for more interesting topics on Software development.